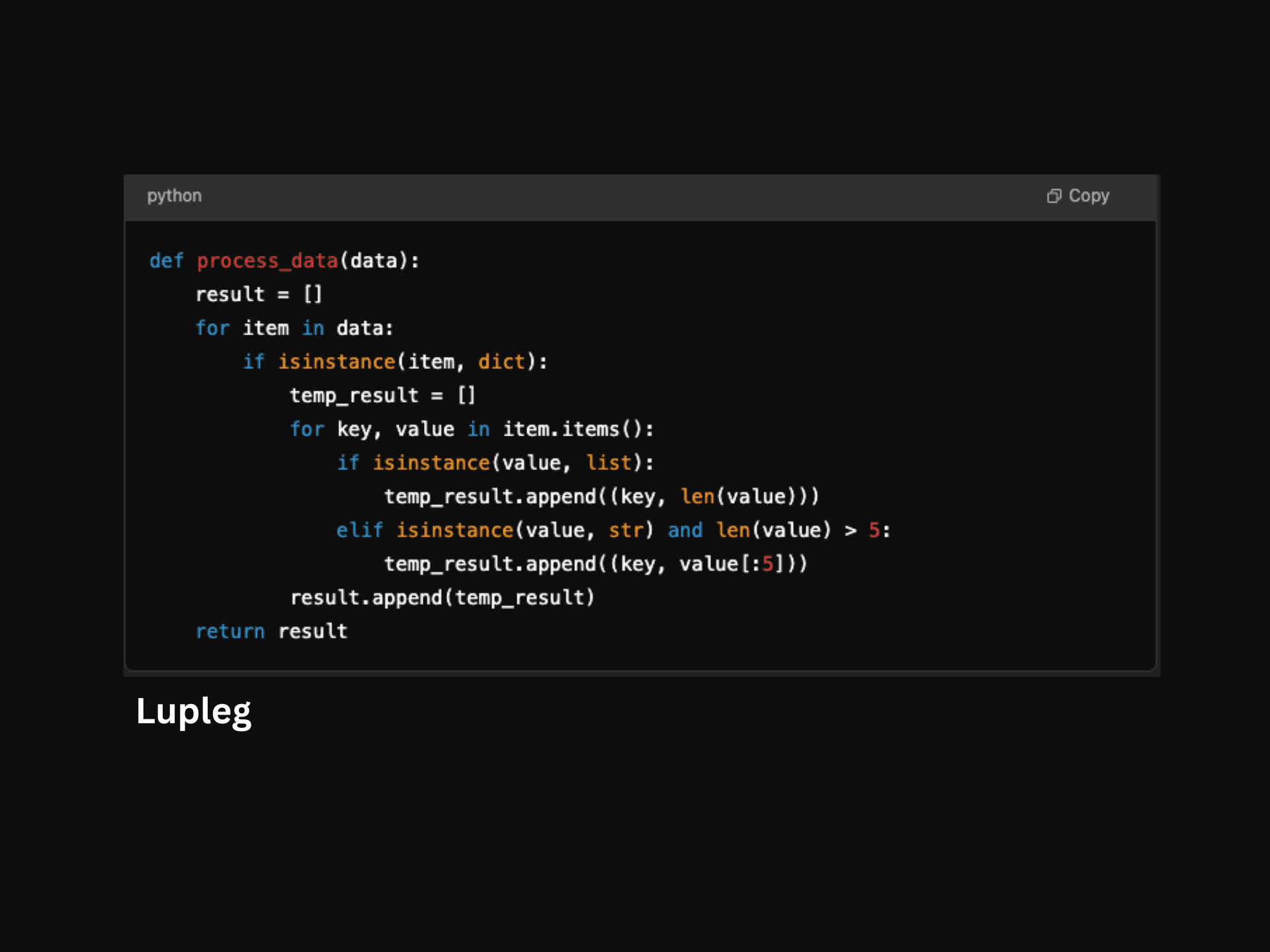
Python has become one of the most popular programming languages due to its simplicity, readability, and versatility. However, to truly harness the power of Python and develop maintainable, efficient code, it’s essential to follow best practices. In this article, we will explore key Python best practices that will help you write clean, effective, and scalable Python code.
## 1. Follow PEP 8 - Python’s Style Guide
PEP 8 (Python Enhancement Proposal 8) is the official style guide for Python code. Following PEP 8 ensures that your code is consistent, readable, and easy for other developers to understand. Some key principles of PEP 8 include:
Naming conventions: Use lowercase for variables and functions, and capitalize class names using the "CapWords" convention.
Indentation: Use 4 spaces per indentation level. Avoid mixing tabs and spaces.
Line length: Limit all lines to a maximum of 79 characters. This improves readability on different screen sizes and when printing or displaying the code.
Adhering to PEP 8 reduces the chances of introducing unnecessary complexity into your code, especially when collaborating with other developers.
## 2. Write Meaningful Variable and Function Names
The clarity of your code largely depends on the names you choose for variables, functions, and classes. Always aim for meaningful, descriptive names that make the code easier to understand. Good names should convey the purpose or behavior of the item in question.
For example, avoid using vague names like x or temp in most situations. Instead, use names that describe their role in the program, such as user_name, total_price, or calculate_discount().
The more expressive your naming, the less documentation you need. Properly named code is self-explanatory, making it easy to follow for future developers or even yourself when revisiting old projects.
## 3. Keep Functions Small and Focused
Functions should perform one task or operation, and that task should be clearly defined. Large functions with multiple responsibilities can be difficult to maintain and debug. Aim to keep your functions small and focused on a single responsibility, adhering to the Single Responsibility Principle.
Smaller functions also increase the reusability of your code. If you make a function too general or complicated, it might become difficult to reuse it in other contexts. By keeping your functions narrowly focused, you will be able to more easily build and modify your programs.
## 4. Avoid Hardcoding Values
Hardcoding refers to embedding fixed values directly in your code. While it might be convenient in the short term, hardcoding reduces flexibility and makes the code difficult to maintain. If a value changes, you’ll have to search through the entire codebase to update it.
Instead of hardcoding, consider using variables, constants, or configuration files to store values that may change. This helps keep your codebase flexible and adaptable to future modifications.
## 5. Handle Exceptions Properly
Python provides robust exception handling mechanisms that allow you to anticipate and manage errors. Instead of letting your program crash when an error occurs, proper exception handling helps you gracefully recover from unexpected situations and provides informative error messages for debugging.
## When handling exceptions:
Be specific about the types of exceptions you catch.
Avoid catching generic exceptions (such as Exception) unless absolutely necessary.
Include meaningful error messages to help identify the problem when an exception occurs.
By anticipating and managing exceptions effectively, you improve the stability and user experience of your programs.
## 6. Use List Comprehensions Wisely
List comprehensions are a concise and readable way to create lists in Python. They provide an elegant way to transform and filter data. However, it’s important not to overuse them, especially when the logic within the list comprehension becomes too complex.
Simple list comprehensions can improve both the readability and performance of your code. But if the comprehension becomes difficult to understand, it's often better to stick with a standard for loop. Clarity should always be your priority.
## 7. Avoid Global Variables
Global variables can lead to hard-to-debug issues in your programs. When a variable is accessible from everywhere in the code, it's easy to accidentally change its value, resulting in unexpected behavior.
Instead, prefer passing variables to functions as arguments and returning values from functions. This practice makes your code more predictable and less prone to errors caused by changes to global state.
## 8. Use Python’s Standard Library
Python’s standard library is vast and includes modules for various tasks, such as string manipulation, file handling, data processing, and more. Before implementing your own solution, check whether there’s already a module in the standard library that solves your problem.
For instance, instead of writing your own logic for handling file I/O operations, you can use Python's built-in open() function or libraries like os or shutil. Reusing existing solutions not only saves time but also ensures your code is based on reliable, tested functionality.
## 9. Use Virtual Environments for Dependency Management
When developing in Python, it’s important to manage your project’s dependencies efficiently. Virtual environments (e.g., created using venv or virtualenv) allow you to isolate dependencies for each project, avoiding conflicts between packages.
Using a virtual environment ensures that the libraries you install do not interfere with the global Python installation or other projects. Additionally, tools like pip allow you to easily manage dependencies and share requirements between different development environments.
## 10. Write Unit Tests
Unit testing is an essential part of software development. Writing unit tests ensures that your code behaves as expected and allows you to catch bugs early in the development cycle. Python provides the unittest module for creating and running tests.
Make sure to test each unit of your program independently, including edge cases, to ensure that your code functions correctly. Additionally, testing allows for easier refactoring, as you can rely on automated tests to verify the functionality of your code after making changes.
## 11. Document Your Code
Documentation is crucial for ensuring that others (and future you) can understand and maintain your code. Python offers a great way to document your code using docstrings. A docstring provides an explanation of a module, function, or class and is accessible using the help() function in Python.
Good documentation should describe the purpose, inputs, and outputs of your functions, classes, and modules. By documenting your code, you help make it easier for others to work with your code and improve collaboration across teams.
## 12. Keep Your Code DRY (Don’t Repeat Yourself)
The DRY principle is a cornerstone of writing efficient code. Instead of duplicating code, find ways to generalize it and reuse it in multiple places. Duplicating code leads to maintenance headaches and introduces the potential for inconsistencies.
Refactor your code into functions, classes, or modules that can be reused across your project. This approach improves the maintainability of your code and reduces redundancy.
## Conclusion
Following Python best practices helps you write clean, maintainable, and efficient code. By adhering to established conventions like PEP 8, using meaningful names, handling exceptions effectively, and testing thoroughly, you can ensure that your Python projects are both reliable and scalable. Developing good coding habits early on will not only improve your productivity but will also make collaborating with others and maintaining your codebase much easier in the long run.
By keeping these best practices in mind, you can unlock the full potential of Python, creating high-quality applications that stand the test of time.